Site Visuals in CSS3
Chapter 5
You may have noticed that, with exception of our images, our site does not look very interesting. Black text on a white background isn't very welcoming to our visitors. Let's work on the appearance of our site with CSS (Cascading Style Sheets). Using this language, we can manipulate traits of the site like color, font size, and many other qualities. There is a huge list of possibilities available with CSS.
In the previous sections, we used HTML to describe the content of the site, and divide it into fragments according to their importance. CSS will be responsible for the appearance of our web sites. CSS code can be placed in a separate file with the extension .css and inserted via a special HTML tag. You can also put it directly into the HTML document.
Imagine for a moment, in this abstract example, that we want to construct a house with CSS code, where we choose items such as windows, doors, roofing, walls, gutters and so on. We would want to buy windows of specific sizes, and paint for each of the necessary parts. If we built this house in CSS, one of the many solutions to this task might look like this:
roof {
background-color: green;
}
doors {
background-color: yellow;
width: 100px;
height: 300px;
}
windows {
border: 5px solid brown;
width: 150px;
}
Let's analyze the "roof" block of this code from top to bottom (note that top-to-bottom reading is a rule when reading all types of code, not just HTML and CSS).
roof {
background-color: green;
}
If we translate the code above into normal English, we have chosen an element called "roof" and set the background color to green.
windows {
border: 5px solid brown;
width: 150px;
}
The code above says, "for all windows, set the following: a frame (border) with a width of 5 pixels (5px), marked by a continuous line (solid) color (brown). Also, the window itself should have a (width) of 150 pixels (150px).
You might have noticed a recurring pattern in the code. On the first line, we write the name of the element (termed "selector"), and then define the appearance of that element between bracket.
The template has the basic structure shown below:
selector {
property_name: property:value;
}
This type of construction is a typical CSS rule. The rule consists in turn of a selector (everything before the first bracket) followed by a list of properties that you write between the brackets.
There are various way to specify exactly what we want to design. Let's say that we only wanted to specify design for windows on the ground floor. What then? We could write something like:
ground floor window {
border: 5px solid brown;
width: 150px;
}
The result is that only the selector has changed. Instead of "window {" we have specified "ground floor window {"
This code reads from left to right like "find the ground floor, and then find its window and set the following values." If we put a sub-selector under "ground floor windows" like:
adjacent wall windows {
Then we're telling the browser: "find the ground floor window, windows next to it, and fill them with the following values," and so on. If you remember the analogy in which we talked about nested HTML tags as the children and parents, this is the same concept, elements nested within other elements.
Unfortunately a browser can't quite build a house, but our example tells us how CSS works. This analogy is useful because as we code, we're not always able to see the changes. But we can think of paragraphs (<p>) as windows, and doors as the header (<h1>), etc.
It would look like this:
p {
}
h1 {
}
Let's apply what we've learned in this analogy to our example and use the same ideas to add a little color and life to the Justin Beaver article.
Recall that our code looks like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Justin Beaver fascinated by HTML</title>
</head>
<body>
<article>
<header>
<h1>Justin Beaver: Ever since I learned HTML, my life has made a complete 180</h1>
<p>Posted by: Damian Wielgosik</p>
</header>
<p>Justin Beaver confessed something that even his greatest fans would have never expected of the skilled musicians and lyricist. The young rock-and-roller admitted that since he typed his first title tag, his life became easier. It has been reported by those surrounding the Canadian that Beaver's private mentors, Ryan Loseling and Nicolas Crate, often walk around Los Angeles disputing what a great tool the HTML validator is.</p>
<figure>
<img src="cat.jpg" alt="Justin Beaver's cat is pleased">
<figcaption>Justin Beaver's happy cat</figcaption>
</figure>
<p>Beaver has already created some websites and does not intend to stop there. <q>I will probably have a song about HTML on my next album,</q> - the artist added.
</p>
</article>
</body>
</html>
Recall the analogy of building a house. Instead of building doors, windows, etc., we are dealing with elements like <article>, <p>, <header>, <body>, <figcaption> and so on. These tags build the page and now CSS will help to give them style.
I've prepared a screenshot on the next page so you can see how our modifications will change the resulting website.
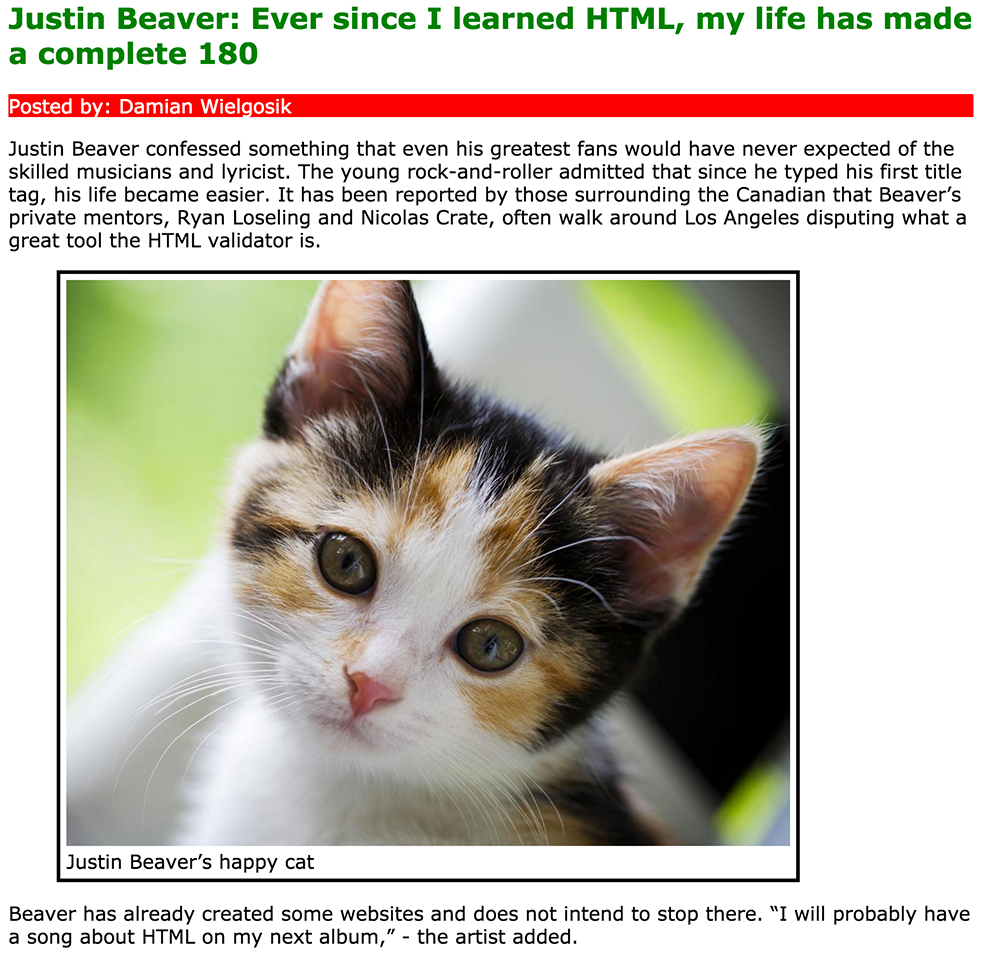
As you can see, much has changed. We've added simple colors, backgrounds, changed the font style and so on. Let's proceed step-by-step for how to accomplish the effects in the image above.
The first step is to save the entire HTML code into a separate file. For me it's called article.html. Then create a separate file in which we keep our CSS rules. Let it be main.css.
The files look like this on my computer:

We can now try to open article.html in a browser and the main.css file using a text editor. I recommend Sublime Text Editor or TextMate. After each change made in main.css, we can refresh the browser the page in order to update its appearance.
We now need to open the article.html in a browser, and load code from the main.css file. This is done through the tag <link> in the <head> in the HTML code. Just add a tag like this in the head:
<link rel="stylesheet" href="main.css">
The "href" attribute indicates where the file is located. "Stylesheet" tells us that it is a CSS style sheet.
Okay, to start making changes to visual appearance in CSS, let's try to find the right selector for the heading, similar to the code for windows and walls.
h1 {
}
Here we are! It is here that we can tell the browser "for all elements in <h1>, apply the following appearances." Note that the braces are currently empty. Let's try to tell it that we want the heading text to be green. We'll apply the property "color" and set it to "green."
h1 {
color: green;
}
The operation for this rule is explained in the diagram below:
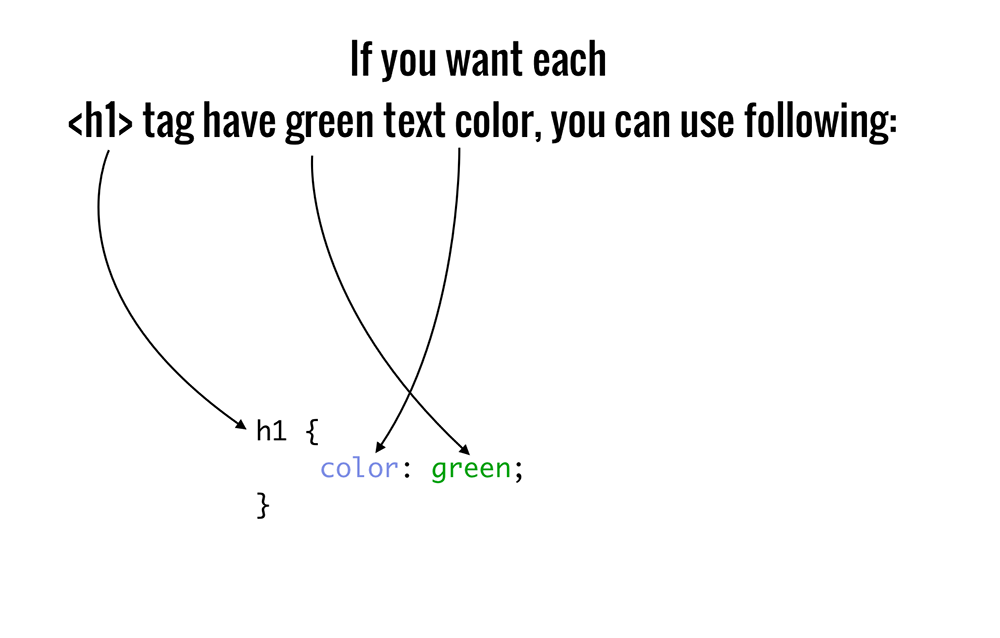
Let's check out how our page looks after the changes!

Yes! The title is indicated in green.
Now we want to address the next section with information about the author. Let's say we want the text in white with a red background as shown a few pages ago. This is the current HTML code:
<article>
<header>
<h1>Justin Beaver: Ever since I learned HTML, my life has made a complete 180.</h1>
<p>Posted by: Damian Wielgosik</p>
</header>
Let's use CSS, and find the appropriate selector ("p {" ) and try to give it a background red color and a white color text:
p {
background-color: red;
color: white;
}
Our main.css code should currently look like this:
h1 {
color: green;
}
p {
background-color: red;
color: white;
}
As you can see, we add a rule one under the other. Time to see how it looks now our website...

Oops, that's not quite right. It seems all the other paragraphs have also been changed to have the new background and text color. It's a problem with our code, because we used the following:
p {
background-color: red;
color: white;
}
What we actually told the browser is to "find all <p> elements and apply changes." However, we only wanted to change the paragraph in the header line of the article.
We now need to modify the code so that the above selector to only apply to the <p> in <header>, which is a "child" of the <article>. The code should reflect this hierarchy:
article header p {
background-color: red;
color: white;
}
Let's see the effect of these changes.

Much better! It seems we were able to target the correct paragraph. But how did this happen? Well, we used the above code to tell the browser to know which tags the CSS selector should target. We do this by examining the HTML code and finding all the tags which should match the selector. In our case, we had nested tags of <article>, <header>, and <p>, so the CSS selector "article header p" let's us specify exactly where the changes will be applied.
Let's move on to the image in the article.
The dimensions of this article, let's say, should be 600 pixels wide. And remember that our corresponding HTML tag for the image is <figure>. Let's specify our CSS code to reflect this:
article figure {
width: 600px;
}
With this code, every <figure> in the <article> tag will have a width of 600 pixels. Note that the "article" distinction would be helpful if we had multiple images throughout the blog post and wanted to specify different criteria for each. But since we only have one image, let's move on to the border code:
article figure {
width: 600px;
border: 3px solid black;
}
Here we've added a property called "border." After the colon, we specify the width of the border (3 pixels), and the style of the border "solid" with the color "black."
Let's see how it looks:
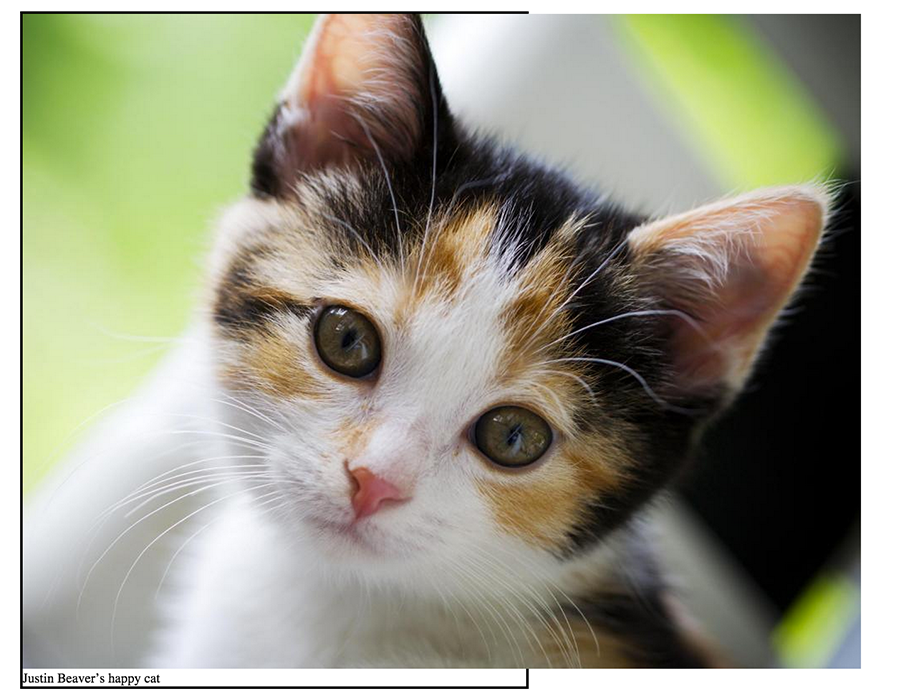
It looks like we have a problem. While the border is displayed with the correct style and color, the image displays beyond our 600 pixels. This is because we established the width of the element <figure>, but the <img> tag does not have any fixed width and thus keeps its original size. It would be nice if the image took 100% of the width of its parent <figure>. This is coded very simply:
article figure img {
width: 100%;
}
It now looks like this:
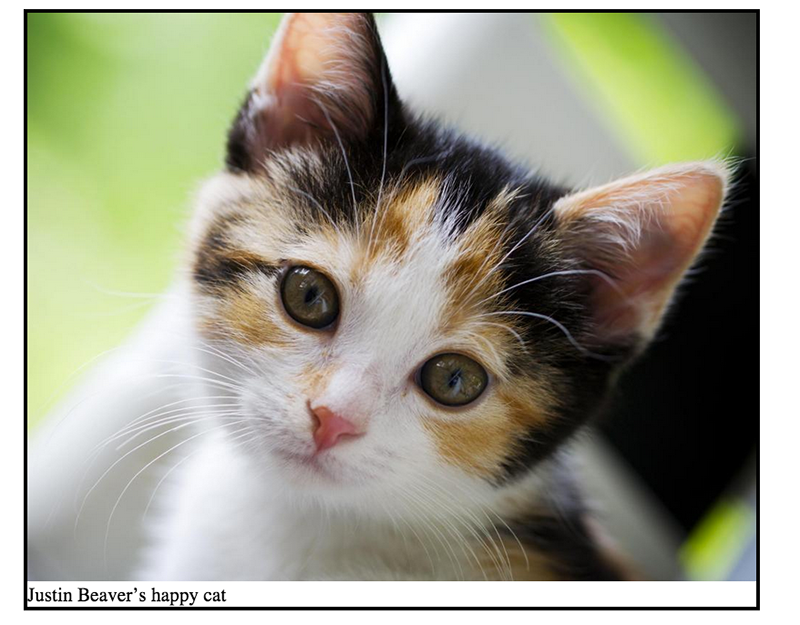
It would be nice to add some "padding" or space between the border and image. We do this by adding the property "padding." We can modify the code as follows:
article figure {
width: 600px;
border: 3px solid black;
padding: 5px;
}
The result:
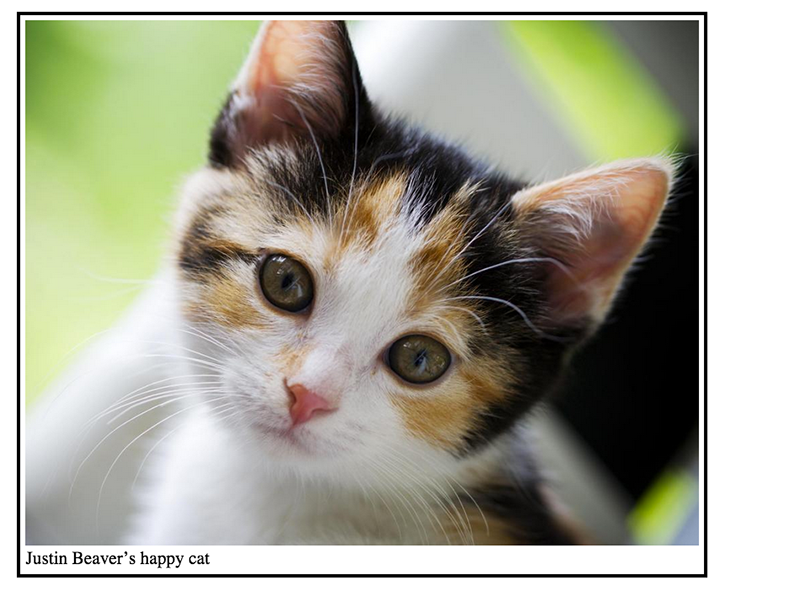
You can try yourself to modify the value of the "padding" and see how the white gap changes between the picture and the border.
Our page is looking good now, but we're not yet finished. The current paragraph text extends almost the entire width of the browser window which isn't very readable. Perhaps it would be fitting to somehow reduce the width of the text? Maybe limit it to 800 pixels?
Let's choose a special CSS selector for this:
article {
width: 800px;
}
That's better.
Now what about font? If you look at the original image of our site, we have a slightly different font. Just as you can edit font styles in Microsoft Word, you can edit them in CSS too. In order to specify font, you want to add this property to the highest tag so that it applies to all text within that tag. For example, we'll set the font as a property for <body>, so that every element below <body> will have this setting. In the picture, I used a font called Verdana.
Let's try to apply it:
body {
font-family: Verdana;
}
You can see the differences by deleting this line or changing the font-family to a different style. For the header, paragraphs, etc. the browser will display everything in Verdana.
Finally, our code in the main.css file should look like this:
body {
font-family: Verdana;
}
article {
width: 800px;
}
article header h1 {
color: green;
}
article header p {
background-color: red;
color: white;
}
article figure {
width: 600px;
border: 3px solid black;
padding: 5px;
}
article figure img {
width: 100%;
}
In general, it's good practice to start your code with the most general selectors and move into more complex ones. I started from body, followed by article, and so on, going from top to bottom. The higher the detail, the lower it sits in the list.